거누의 개발노트
[파이썬] 297. Serialize and Deserialize Binary Tree - 리트코드 본문
반응형
문제
이진 트리를 직렬화 및 역직렬화하는 알고리즘을 설계합니다. 직렬화/역직렬화 알고리즘이 작동하는 방식에는 제한이 없습니다. 이진 트리를 문자열로 직렬화할 수 있고 이 문자열을 원래 트리 구조로 역직렬화할 수 있는지 확인하기만 하면 됩니다.
입력
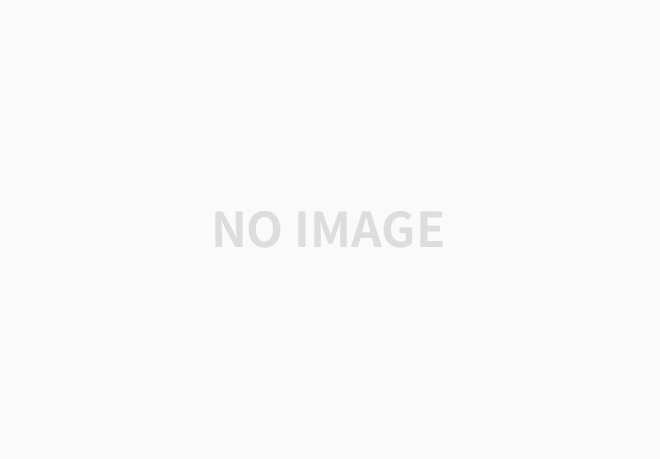
Input: root = [1,2,3,null,null,4,5]
출력
Output: [1,2,3,null,null,4,5]
작성한 코드
# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Codec:
def serialize(self, root):
q = collections.deque([root])
rtn = ['@']
while q:
node = q.popleft()
if node:
rtn.append(str(node.val))
q.append(node.left)
q.append(node.right)
else:
rtn.append('@')
return '#'.join(rtn)
def deserialize(self, data):
print(data)
if data == '@#@':
return None
nodes = data.split('#')
root = TreeNode(nodes[1])
q = collections.deque([root])
idx = 2
while q:
node = q.popleft()
if nodes[idx] != '@':
node.left = TreeNode(int(nodes[idx]))
q.append(node.left)
idx += 1
if nodes[idx] != '@':
node.right = TreeNode(int(nodes[idx]))
q.append(node.right)
idx+= 1
return root
# Your Codec object will be instantiated and called as such:
# ser = Codec()
# deser = Codec()
# ans = deser.deserialize(ser.serialize(root))
처음에는 풀지 못했지만 풀이를 보니까 그렇게 어려운 문제는 아닌것 같다는 생각이 들었다.
잘 이해해서 내걸로 만들면 된다.
다른 사람들에게 설명해주는데 코드가 꼬여서 설명하려고 했던 말들이 잘 안나왔다.
역시 설명도 많이 해봐야겠다. 복습 할 때는 다른 사람한태 설명한다고 생각하고 다시 풀어봐야겠다.
https://leetcode.com/problems/serialize-and-deserialize-binary-tree/
Serialize and Deserialize Binary Tree - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
반응형
'코딩테스트' 카테고리의 다른 글
[파이썬] 1337. The K Weakest Rows in a Matrix - 리트코드 (0) | 2022.05.25 |
---|---|
[파이썬] 1464. Maximum Product of Two Elements in an Array - 리트코드 (0) | 2022.05.25 |
[파이썬] 700. Search in a Binary Search Tree - 리트코드 (0) | 2022.05.24 |
[파이썬] 938. Range Sum of BST - 리트코드 (0) | 2022.05.24 |
파이썬 알고리즘 인터뷰 - 이진 트리 반전 (0) | 2022.05.24 |